Logic
Overview
Logic blocks are about yes/no questions. Is this project item a sequence? Has this clip been used in a sequence at least once? In programming languages, these kinds of questions can be formulated with (boolean) logic and the answer to the question is either the value true
(for yes) or false
(for no).
In Automation Blocks, logic values have pointed edges - in contrast to texts or numbers, which have rounded edges:

So whenever you see these pointed shapes, you know that a logic value (or yes/no question) is expected at that point.
If
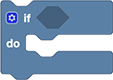
The if block is the the most essential block in the logic category. Whereas all other logic blocks are about formulating logic questions, the if block is about what should actually be done if the answer to the question is yes (or what else should be done when the answer is no).
At the top of the if block, you need to insert a logic value, aka a yes/no question, and the blocks you plug inside the if block are only executed if the answer to this question is yes (i.e. true
). This example writes the sequence has markers
to the console if the active sequence has at least one marker (and otherwise does nothing):

If ... Else
When you click on the blue cogwheel icon on the top left of the if block, you can also add an else section too. The else section will only be executed if the answer to the question is no (i.e. false
). Let's extend our example so it outputs the sequence has NO markers
if the sequences does NOT have at least one marker:
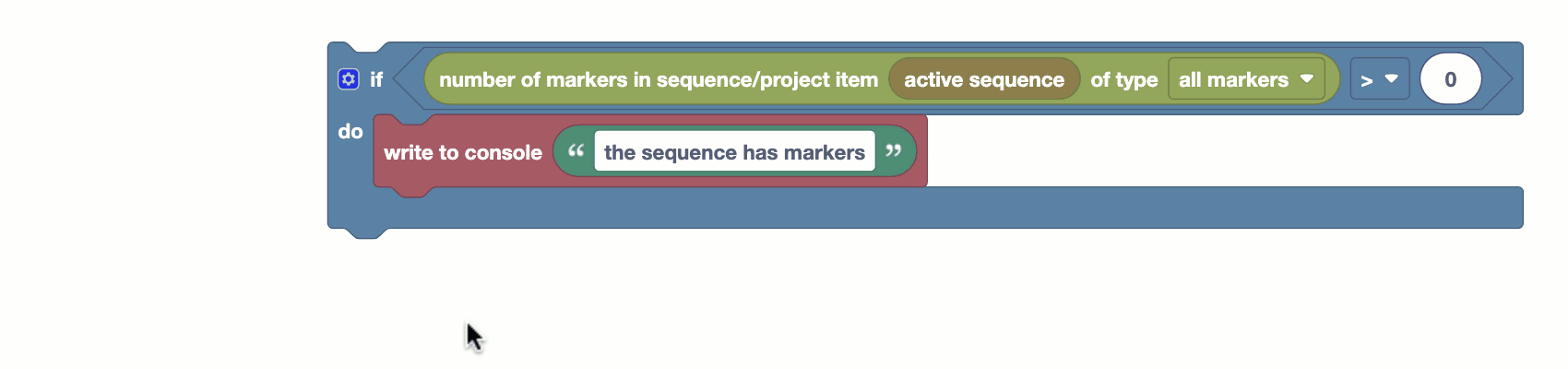
If ... Else If ... Else
Finally, you can have an if block that asks multiple questions by adding else if sections to the block. When the answer to the first question is false
(and only in that case), it does not go to the else block immediately, but first asks the second question. Here is an example:
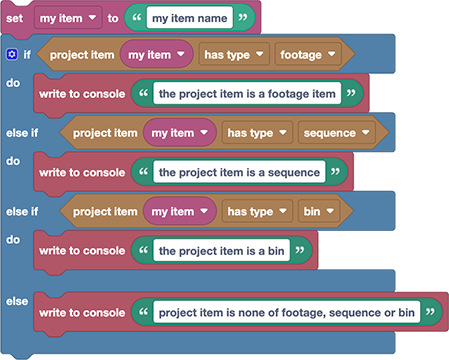
This example checks the type of the project item with name my item name
. The if block executes exactly one of the "write to console" blocks in order to state what type the project item actually is.
Compare
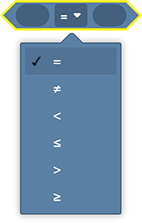
This block can compare two numbers. Use it to ask questions like "Are the numbers equal?" or "Is the first number greater than the second number?".
Example: Has Footage been used?

Here we use the Compare block to ask if a footage item has been used at least once ("Is the number of times the footage item has been used greater or equal to one?"):
And/Or
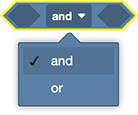
With this block you can combine two questions into one. If you choose "and", the result is only true
if both questions are answered with true
. For "or" the result is true
if at least one of the two questions is answered with true
.

This example returns true
if either the video or the audio of the footage item (or both audio and video) has been used at least once.
Not

This block turns a true
into a false
and vice versa.
Example: End of Textfile

The End of File block returns true
if the script has read the entire contents of the text file already. If you plug it into a Not block, it returns true
if there is still content left to read.
Boolean (Logic Value)
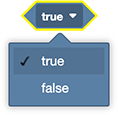
This block always returns true
or always returns false
(depending on which of the two you have chosen in the dropdown).
Checkbox

This block is identical to the normal boolean block, just with a different visual representation. If the checkbox is checked, it has the value true
and otherwise false
.
Null

The JavaScript value null, which represents the intentional absense of any object value.
You can also use Null directly in a logic test (as the condition of an if block, for example). In a logic test, Null will behave like false
whereas any other object behaves like true
. For example, see the Ternary Test below.
Ternary Test
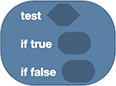
This block is similar to an if...else statement. The difference is the following: with the if...else block, you can plug in two block stacks (where one block stack is executed when the condition is true
and the other when the condition is false
). In the Ternary Test you plug in two values instead of two block stacks and the first value is returned if the condition is true
and the second is returned if the value is false
. In other words, this is a mini-version of the if....else block that is just about calculating a single value instead of executing arbitrary blocks.
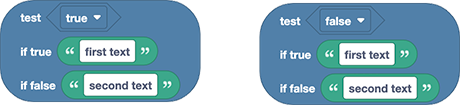
In this example, the block on the left returns first text
whereas the block on the right returns second text
.
Example: null and markers
This script prints on the console first sequence marker is at 5 seconds
if the active sequence has its first marker at that time and if the active sequence has no marker, it prints sequence has no marker
instead.
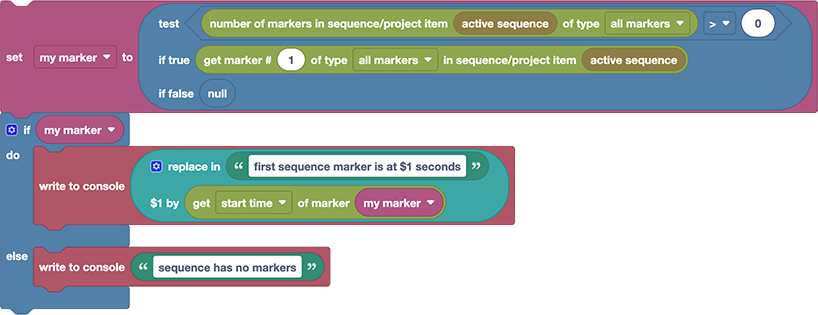
In the first line, we save the first marker of the active sequence in the variable my marker
. If the sequence has no marker, we give the variable the value null
instead, to indicate that there is no such marker object.
When we want to use the marker, we need to first check if the marker actually exists or is null
. To do this, we test the my marker
variable in an if block. Since null
is equivalent to false
(whereas any other object, like a valid marker, is equivalent to to true
), the first write to console block is only executed if a marker exists, and otherwise the second one only.